Sometimes, you really need to special case something, but doing so is ugly, and not going to pass code review easily. Justifying and testing these edge cases might be really difficult, and it'd be better if you could just slip them in without anyone noticing. Luckily, structured control flow gives us the tools needed to hide edge cases in plain sight. First, observe the following pseudo-code:
string data;
if (auto result = SerializeData(value); result.ok()) {
data = result.value();
} else {
data = "-";
}
Now consider the following: it might be really, really hard to get SerializeData
to return an error result, and even if you could, it might be really, really hard to handle it in a more meaningful way than just simply swallowing the error. Maybe, then, it would make sense to log it, so that it doesn't get lost:
string data;
if (auto result = SerializeData(value); result.ok()) {
data = result.value();
} else {
data = "-";
LOG(ERROR) << "Unexpected error in SerializeData: " << result.error();
}
That's all good and well, but wow, it really draws attention to the fact that you're not actually handling this error meaningfully. Even worse, it adds another line of uncovered code, when we'd rather have none.
Thankfully, we don't need an else
branch. We already have an if
branch, after all. So we can just eliminate our else
case by making sure all of the side-effects it would have are simply already in effect in the case where the if
isn't taken. In this case:
string data = "-";
if (auto result = SerializeData(value); result.ok()) {
data = result.value();
}
Nice! Now we're really not drawing any attention to the edge case, and line coverage reads 100%.
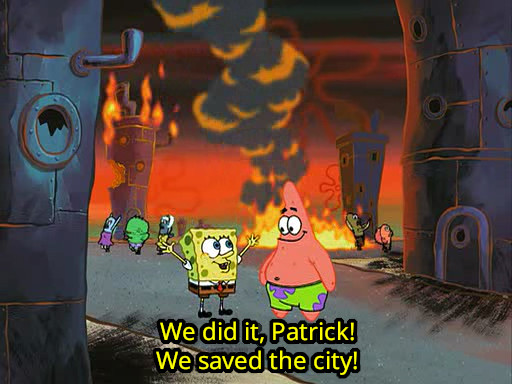
Of course, in this case it's so obvious as to be trivial, but it's often possible to restructure your code around this if you really want to. I do so for innocuous reasons just to clean up the control flow.
Does this trick work in real life? Well, since I would never stoop to tricks like these, I can neither confirm nor deny the efficacy of this technique. However, if you happen to read this from a Google workstation, please do not check my CL history.